A List of Git Commands
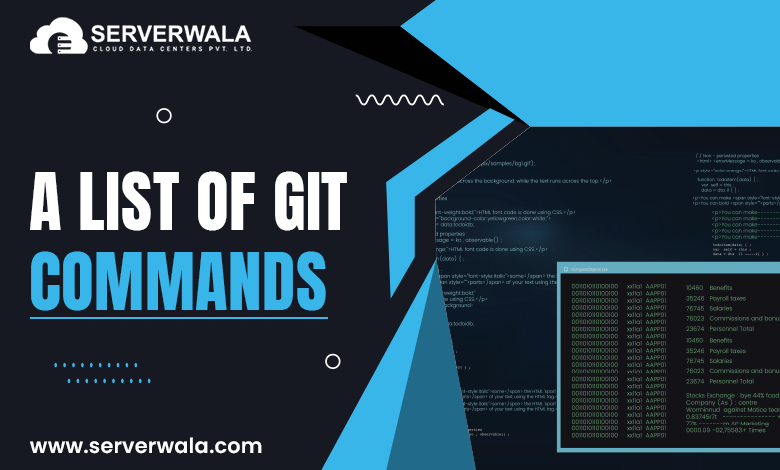
Introduction
Software development is a continuous process of writing, modifying, and improving code. Without proper version control, managing changes becomes chaotic, especially in collaborative projects. This is where Git, the most widely used distributed version control system (DVCS), plays a crucial role.
Git enables developers to track every modification done to a codebase, ensuring that past versions are never lost. It provides a structured way to work on different features simultaneously, test new functionalities, and merge updates without conflicts. It does not matter if you are a single developer or part of a large team, Git helps maintain an organized and error-free development process.
With Git, you can:
- Create and manage multiple versions of your project through branches.
- Respond to earlier versions if anything goes wrong.
- Collaborate efficiently by syncing your code with remote repositories such as GitHub, Bitbucket, etc.
- Work offline since Git operates locally, syncing only when necessary.
- Boost security by tracking every modification, ensuring accountability and transparency in projects.
Git is fast, scalable, and designed for efficiency. It supports teams working on open-source projects, enterprise software, mobile applications, and even AI models. Entities such as Microsoft, Netflix, etc., depend on Git for managing their complex codebases.
With this guide, you will apprehend the fundamental Git commands used in everyday development. You will learn how to set up Git, configure your settings, manage files, create branches, and interact with remote repositories.
Let’s dive in and explore how Git can enhance your coding experience!
Git Setup
Before you can begin employing Git, you are required to install and configure it properly. This ensures that you can track modifications, work together on projects, and handle code effectively. Follow these steps to set up Git on your system:
Step 1: Install Git
Git is accessible for Windows, macOS, & Linux. To install it:
- Download the latest version from the official Git website: git-scm.com.
- Run the installer & follow the setup instructions. Windows users should ensure that “Git Bash” is included in the installation.
- Restart your terminal (Command Prompt, Git Bash, or Terminal) after installation to apply changes.
Step 2: Verify the Installation
To confirm that Git is installed aptly, open a terminal and run:
git --version
If the installation is successful, this command is going to show the installed Git version. If not, try restarting your system or reinstalling Git.
Step 3: Set Up Your User Identity
Before you start using Git, you are required to configure your name as well as email. This data will be linked to every commit you make.
Execute the given below commands:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
These settings apply to all Git projects on your system. If you desire to employ different identities for different repositories, remove the –global flag and operate the command inside a particular repository.
Step 4: Initialize a New Repository
For constructing a new Git repository in your project directory, navigate to the folder in your terminal and run:
git init
This command initializes an empty Git repository, allowing you to start tracking modifications in that directory. Git will construct a hidden .git folder where it stores all version history and metadata.
Step 5: Clone an Existing Repository
If you’re contributing to an existing project, you can copy the repository to your local machine using:
git clone <repository_url>
Replace <repository_url> with the actual URL of the repository from GitHub, GitLab, or Bitbucket. This downloads the entire project along with its commit history.
Since Git set up is done, you can start managing your code effectively!
Git Configuration
Once Git is installed, configuring it properly ensures a consistent and efficient workflow. Git provides various customization options to suit your development preferences.
Step 1: Check Your Current Configuration
To see all the Git settings on your system, use:
git config --list
This displays a list of all configurations, including user identity, default text editor, merge behavior, and color settings.
Step 2: Set a Default Text Editor
Git sometimes opens a text editor when writing commit messages or tackling merge conflicts. You can specify your preferred editor:
For VS Code:
git config --global core.editor "code --wait"
For Vim:
git config --global core.editor "vim"
For Nano (a simple built-in editor):
git config --global core.editor "nano"
The –wait option ensures Git holds on for you to end editing before moving further.
Step 3: Enable Color Output
To make Git’s output more readable, enable color-coded messages:
git config --global color.ui auto
This makes it easier to differentiate between added, modified, and deleted files when running Git commands.
Step 4: Set Default Branch Name
By default, Git names the first branch master. Many modern projects prefer using main as the default branch name. You can set it globally:
git config --global init.defaultBranch main
Step 5: Configure Auto-Correct for Mistyped Commands
Git can suggest the correct command if you make a typo:
git config --global help.autocorrect 1
With this setting, if you type git stats instead of git status, Git will automatically correct it.
Step 6: Configure Credential Storage
To avoid re-entering your credentials every time you interact with a remote repository, enable credential caching:
For Mac/Linux:
git config --global credential.helper cache
For Windows:
git config --global credential.helper wincred
This keeps your credentials securely, making authentication seamless.
Step 7: Configure Aliases for Shorter Commands
Aliases enable you to save time by constructing shortcuts for often employed commands. For instance:
git config --global alias.st status
git config --global alias.co checkout
git config --global alias.br branch
git config --global alias.cm "commit -m"
Now, rather than typing git status, you can easily use git st.
Managing Files
Git helps you track changes in files across different stages. Every file in your repository exists in one of three states:
- Untracked – The file is new and not yet being tracked by Git.
- Staged – The file has been modified and added to the staging arena, ready for commit.
- Committed – The file is saved in Git’s history.
Understanding how to manage files effectively ensures a hassle-free version control workflow. Below are essential Git commands for handling files.
1. Check the Repository Status
To view the current state of your operating directory & staging area, run:
git status
This command displays modified, staged, and untracked files, helping you decide what actions to take next.
2. Append Files to the Staging Area
Prior to committing, you need to stage changes using git add.
For staging a particular file:
git add filename
To stage all changed & new files at once:
git add .
Using . ensures that every modification in the operating directory is staged. However, you must be conscious when employing this command, as it includes all modifications.
3. Commit Changes
As soon as files are staged, you are required to commit them to save the modifications in Git’s history.
git commit -m "Descriptive commit message"
A good commit message must clearly explain what modifications were happened. This supports in tracking advancement and understanding past modifications.
4. Remove a File from Tracking
If you want to stop tracking a file but keep it in your working directory, use:
git rm --cached filename
To discard a file completely from the repository & the operating directory, use:
git rm filename
This deletes the file from the project. To apply the removal, commit the changes:
git commit -m "Removed filename"
5. Move or Rename a File
To rename a file or move it to a different directory within the repository, use:
git mv oldname newname
This command works like the mv command in Linux and ensures that Git tracks the rename operation properly. After renaming or moving, commit the changes:
git commit -m "Renamed oldname to newname"
Effectively managing files in Git allows you to maintain a clean, organized, and structured codebase.
Working With Git Branches
Git branches are essential for parallel development, allowing multiple developers to operate on varied attributes or fixes without manipulating the principal codebase. By using branches, you can experiment, isolate changes, and merge updates when ready.
1. View All Branches
To list all branches in your repository, use:
git branch
The active branch is marked with an asterisk (*).
2. Construct a New Branch
To create a new branch without switching to it:
git branch branch-name
This creates a branch but keeps you on the current one.
3. Switch to Another Branch
To move to a different branch, use:
git checkout branch-name
This updates your working directory to reflect the latest state of the selected branch.
4. Create and Switch to a New Branch in One Step
Instead of running two commands to create and switch branches separately, you can do both at once:
git checkout -b branch-name
This is useful when you wish to begin operating on a new attribute immediately.
5. Merge One Branch into Another
As soon as your feature is done, you can merge it into the main branch (typically known as main or master). First, switch to the main branch:
git checkout main
Then, merge the changes from another branch:
git merge branch-name
Merging combines the work from branch-name into main. If there are conflicts, Git will as you to tackle them before finishing the merge.
6. Delete a Branch
Once a feature branch is merged and no longer needed, you can delete it:
git branch -d branch-name
If the branch has unmerged changes and you are sure you want to delete it, use:
git branch -D branch-name
This forces the branch deletion, even if it contains unmerged changes.
7. Work with Remote Branches
To list remote branches:
git branch -r
To fetch and track a remote branch locally:
git checkout -b local-branch-name origin/remote-branch-name
To push a newly created branch to the remote repository:
git push -u origin branch-name
Git branches are a powerful tool for organizing code changes, enabling efficient collaboration, feature isolation, and version control. Mastering branch management improves workflow and makes teamwork seamless.
Making Changes
Once you modify files, Git tracks them. Use these commands to manage changes:
View changes in a file:
git diff filename
View changes between commits:
git diff commit1 commit2
View commit history:
git log --oneline --graph --decorate --all
Undoing Changes
Made a mistake? Git helps you roll back.
Unstage a file:
git reset filename
Discard all local changes:
git checkout -- filename
Revert a commit:
git revert commit-id
Reset to a previous commit:
git reset --hard commit-id
Be careful—this removes all changes after the commit.
Rewriting History
Git permits you to alter past commits, which is helpful when you need to correct mistakes, improve commit messages, or clean up your commit history before letting others access it. However, rewriting history should be done with caution, especially if changes have already been pushed to a shared repository.
1. Amend the Last Commit
If you need to modify the most recent commit, such as updating the commit message or adding forgotten changes, use:
git commit --amend -m "Updated commit message"
This replaces the last commit with a new one while keeping the same parent. If you have additional files to include in the last commit, stage them first using git add, then run the amend command.
Warning: If you’ve already pushed the commit, use git push –force to upgrade the remote branch. However, this can overwrite history and impact team collaboration.
2. Rewriting Multiple Commits with Interactive Rebase
To reorder, modify, squash, or delete previous commits, use interactive rebase. For example, to edit the last three commits, run:
git rebase -i HEAD~3
This opens an interactive editor where you can:
- Pick (keep commits as they are)
- Reword (edit commit messages)
- Squash (merge multiple commits into one)
- Drop (remove commits)
After making the necessary changes, save and close the editor. Git will replay the commits with your modifications.
3. Undo a Specific Commit Without Losing Changes
In case you need to undo a commit but have the modifications stay in your operating directory, use:
git reset --soft HEAD~1
This moves the latest commit back to the staging area, allowing you to make adjustments and recommit.
For completely removing a commit without keeping the changes, use:
git reset --hard HEAD~1
Caution: This permanently deletes the commit and its changes. Use with care.
If a commit has already been pushed and needs to be undone without altering history, use git revert:
git revert commit-hash
This creates a new commit that undoes the changes made by the specified commit, keeping history intact.
Mastering history rewriting helps keep your commit log clean, organized, and professional. However, use these commands carefully, especially when working in a collaborative environment.
Remote Repositories
Remote repositories allow multiple developers to collaborate by pushing and pulling code from a shared source. They enable version control across different machines and teams, making Git a powerful tool for distributed development.
1. Adding a Remote Repository
To connect your local repository to a remote server, use:
git remote add origin https://github.com/user/repo.git
This command links your local repository to the remote repository at GitHub (or another Git hosting service). The origin name is a conventional alias for the remote repository, but you can change it if needed.
2. Viewing Remote Repositories
To see the list of configured remote repositories, run:
git remote -v
This shows both the fetch as well as push URLs linked with each remote.
3. Fetching Changes from a Remote Repository
Fetching downloads updates from the remote repository while not merging them into your local branch:
git fetch
It is helpful for reviewing new modifications before incorporating them into your working directory.
4. Pulling the Latest Changes
For fetching as well as merging updates from the remote repository into your current branch, use:
git pull origin main
This guarantees your local branch is up to date with the most current modifications from the main branch of the remote repository.
Tip: If you want to automatically rebase instead of merge when pulling, use:
git pull --rebase origin main
This keeps history cleaner by applying your local modifications over the latest remote updates.
5. Pushing Local Changes to a Remote Repository
To upload local commits to the remote repository, use:
git push origin main
This updates the main branch on the remote repository with your local changes.
Note: If pushing to a remote branch for the first time, use:
git push -u origin branch-name
The -u (or –set-upstream) option sets up tracking between the local and remote branches, so future pushes can be done with just git push.
6. Cloning a Remote Repository
For building a local copy of an existing remote repository, use:
git clone https://github.com/user/repo.git
This downloads the entire repository, including its history, and sets up a connection to the remote.
If you want to clone the repository into a specific folder, specify the folder name:
git clone https://github.com/user/repo.git my-project
This creates a local directory named my-project and initializes the repository inside it.
7. Deleting a Remote Repository Reference
If you no longer need a connection to a remote repository, remove it using:
git remote remove origin
This removes the origin reference but does not delete the remote repository itself.
Also Read: What are Git Checkout Tags and How To Use Them?
Git Cheat Sheet PDF
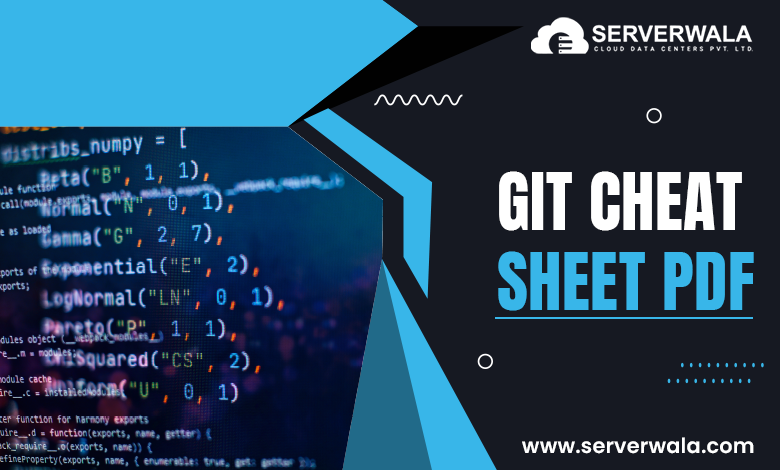
A quick reference guide can be handy. Download a Git cheat sheet from:
Conclusion
Git is more than just a tool for tracking code—it’s the backbone of modern software development. It renders developers the capability to handle projects effectively, integrate seamlessly, and maintain a structured workflow. It does not matter if you are operating on a private project or for big enterprise applications, Git makes code handling smooth and reliable.
By mastering Git commands, you can:
- Enhance productivity by keeping your code well-organized.
- Reduce errors by leveraging branching, commit history, and version rollbacks.
- Improve team collaboration by permitting several contributors to operate on the same work without conflicts.
- Ensure code safety with detailed commit logs and secure backups on remote repositories.
Git’s flexibility makes it essential for developers at all levels. If you’re a beginner, start with the basics—initializing repositories, constructing commits, & pushing modifications. As you acquire experience, explore progressive attributes such as rebasing, cherry-picking, and interactive staging to refine your workflow.
The more you implement, the more efficient you will develop in handling version control. Keep experimenting with Git, use it in real-world projects, and leverage cheat sheets whenever needed.
With Git, coding becomes more organized, productive, and collaborative. Keep exploring, keep improving, and happy coding!