Get Current Date and Time in Python with datetime Module
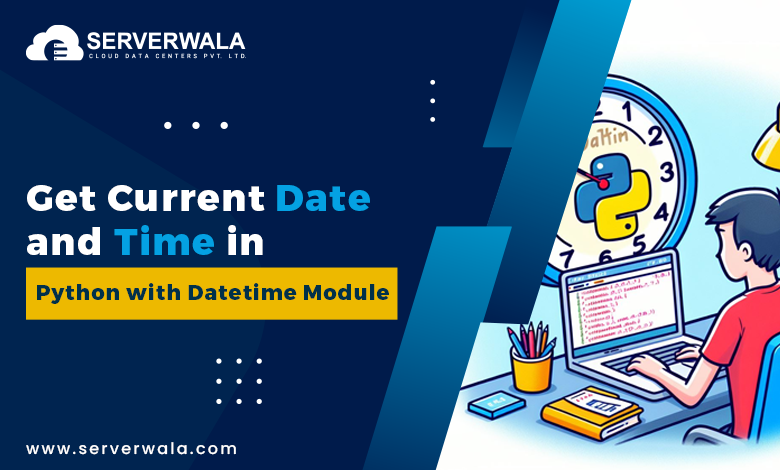
Introduction
Managing date and time in Python is straightforward, thanks to the powerful datetime module. This module is indispensable for a wide range of tasks, such as tracking events, setting reminders, calculating durations, and processing data tied to specific timestamps. Whether you’re building scheduling apps, logging frameworks, or data-driven systems, Python offers versatile methods to fetch, manipulate, and store date and time values.
Python’s datetime module provides flexibility for both basic and advanced requirements. It can handle tasks like formatting dates in different styles, working with time zones, and even measuring time intervals to the millisecond or nanosecond. These capabilities make Python an ideal option for projects that need accurate time management.
With this guide, we will dig deep into several techniques for retrieving and formatting date and time values. You’ll learn how to customize time output to fit your needs and handle critical operations such as working across different time zones and calculating time differences. We’ll also cover how to achieve high-precision timing for performance monitoring or financial systems. These features are essential when building robust systems like logging and monitoring applications, real-time event tracking, and complex scheduling solutions. Python’s date and time tools offer unparalleled flexibility and can streamline workflows, ensuring time accuracy across various applications.
How to get current date and time in Python?
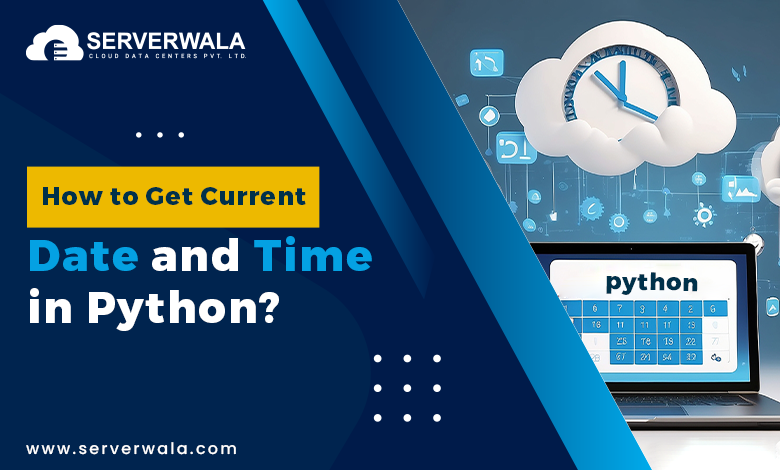
Get Current Date with datetime
To fetch the current date in Python, you can employ the datetime.date.today() method. This function delivers the date in the YYYY-MM-DD format, which is universally recognized and easily parsed.
Following is an instance of how to use it:
from datetime import date
current_date = date.today()
print("Today's Date:", current_date)
This method is invaluable for applications that rely on daily updates, such as generating reports, managing schedules, or setting reminders. For instance, calendar applications use it to highlight the current day, while scheduling systems leverage it to determine deadlines or start dates.
Additionally, you can combine this functionality with string formatting to display the date in a custom format. For example:
formatted_date = current_date.strftime("%B %d, %Y")
print("Formatted Date:", formatted_date)
This will print the date in a more readable format like “January 17, 2025.” Such customization is useful in user-facing applications that need a friendly and intuitive interface.
For businesses, logging the current date is essential for compliance and tracking purposes. By incorporating date.today() into automated workflows, organizations can ensure accurate date stamping for tasks, files, and communications.
Get Date and Time with datetime
If you need both the date and time, the datetime.now() function is the go-to option. It retrieves the current date and time, complete with microseconds for high precision.
Here’s how you are able to use it:
from datetime import datetime
current_datetime = datetime.now()
print("Current Date and Time:", current_datetime)
This function is particularly valuable in scenarios where precise timestamps are critical. For example, in real-time monitoring systems, it helps log exact times for events, making it easier to debug or analyze system behavior. Similarly, in financial applications, the precise timestamp is vital for recording transactions or tracking market activity.
You also have the privilege to format the output to make it even more user-friendly:
formatted_datetime = current_datetime.strftime("%d-%m-%Y %H:%M:%S")
print("Formatted Date and Time:", formatted_datetime)
This converts the date and time into a readable format like “17-01-2025 14:30:15,” which is useful for reports or logs.
For advanced use cases, datetime.now() can be combined with time zone handling to provide region-specific timestamps. By pairing it with libraries like pytz or zoneinfo, you can ensure accuracy in global systems.
Whether you’re building a logging framework, scheduling system, or timestamping tool, datetime.now() ensures your applications remain precise and reliable.
Get Timezone with datetime
Handling time zones is essential for global applications to ensure accurate scheduling and communication. By combining Python’s datetime module with the pytz library, you can retrieve timezone-aware timestamps effortlessly.
Here’s an example of getting the current time in a specific timezone:
from datetime import datetime
import pytz
timezone = pytz.timezone('America/New_York')
current_time_with_timezone = datetime.now(timezone)
print("Time in New York:", current_time_with_timezone)
This feature is critical for applications operating across multiple regions, such as international conferencing platforms or e-commerce systems. For instance, e-commerce platforms use timezone-aware timestamps to provide accurate delivery estimates or to display order times in the user’s local time.
The pytz library supports a wide range of time zones. To list all available time zones, use:
import pytz
print(pytz.all_timezones)
Additionally, datetime.now() can also be paired with the zoneinfo module (introduced in Python 3.9) for timezone handling without external dependencies:
from datetime import datetime
from zoneinfo import ZoneInfo
timezone = ZoneInfo('Europe/London')
current_time = datetime.now(timezone)
print("Time in London:", current_time)
Timezone handling ensures consistency in global operations, whether you’re coordinating meetings, tracking international transactions, or managing user activity logs in different regions.
Get Time and Date Using strftime
The strftime method in Python allows you to customize the format of date and time outputs. This is particularly useful for creating human-readable outputs or meeting specific formatting standards in various applications.
Here’s a basic example:
from datetime import datetime
now = datetime.now()
formatted_date_time = now.strftime("%d/%m/%Y, %H:%M:%S")
print("Formatted Date and Time:", formatted_date_time)
Common Formatting Codes
- %d: Day of the month (e.g., 01 to 31)
- %m: Month as a number (e.g., 01 to 12)
- %Y: Year with century (e.g., 2025)
- %H: Hour in 24-hour format (e.g., 00 to 23)
- %M: Minutes (e.g., 00 to 59)
- %S: Seconds (e.g., 00 to 59)
For example, to format the output as “January 17, 2025, 14:35 PM,” you can use:
formatted_output = now.strftime("%B %d, %Y, %I:%M %p")
Custom formatting is indispensable in applications like reporting tools, log files, and dashboards. A well-formatted timestamp enhances readability and aligns with user preferences or organizational standards.
Advanced use cases include embedding these formatted outputs into file names for easier organization or appending them to log entries to track events systematically. For instance:
log_filename = f"log_{now.strftime('%Y%m%d_%H%M%S')}.txt"
print("Log File Name:", log_filename)
The flexibility of strftime allows developers to cater to diverse requirements, making it an essential tool for date and time customization.
Get the current date and time in Python
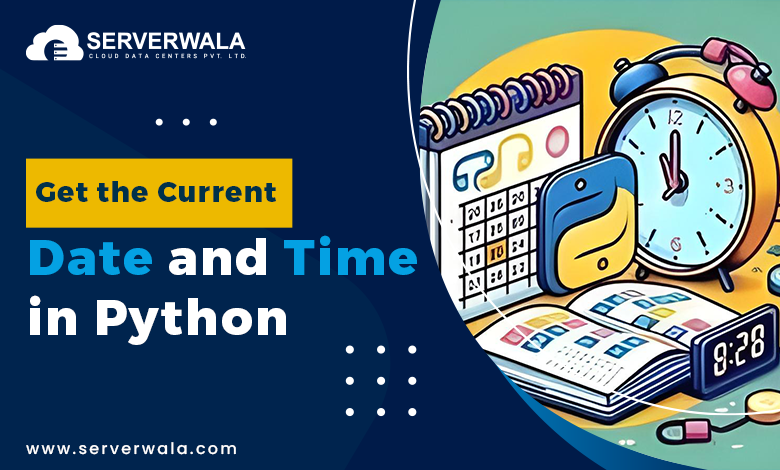
Get Time with strftime
To extract and format only the time portion of the current timestamp, Python’s strftime method is highly effective. This approach allows you to display the time in a human-readable format, tailored to specific needs.
Example:
from datetime import datetime
current_time = datetime.now().strftime("%H:%M:%S")
print("Current Time:", current_time)
How It’s Used
This functionality is particularly useful for applications focused on time-based events, such as alarms, notifications, or reminders. For instance:
- Alarms: Applications can display the current time while setting or modifying alarms.
- Scheduling Systems: Task schedulers use formatted time to trigger events like meetings or system updates at specific hours.
Customizing the Time Format
You can modify the time format to fit different use cases. For example:
12-hour format with AM/PM:
time_in_12_hour_format = datetime.now().strftime(“%I:%M:%S %p”)
print(“12-Hour Format Time:”, time_in_12_hour_format)
- Output: 02:45:23 PM
Display with milliseconds:
from datetime import datetime
current_time_with_milliseconds = datetime.now().strftime("%H:%M:%S.%f")[:-3]
print("Current Time with Milliseconds:", current_time_with_milliseconds)
- Output: 14:45:23.123
Applications requiring precision or readability, such as log files, event triggers, or real-time dashboards, benefit greatly from this flexibility.
Get Time in Milliseconds
For more precise timing, you can use Python’s time module to fetch the number of milliseconds elapsed since the Unix epoch (January 1, 1970). This measurement is widely used in computing to represent time as an integer for easier processing and comparison.
Example:
import time
milliseconds_since_epoch = int(time.time() * 1000)
print("Milliseconds since Epoch:", milliseconds_since_epoch)
Why Milliseconds Matter
Milliseconds are crucial for applications where precision is key:
- APIs: Many APIs rely on timestamps in milliseconds to track and authenticate requests.
- Event Tracking: Platforms like analytics tools and gaming systems use milliseconds to measure and record response times or user interactions.
- Performance Monitoring: In real-time systems, milliseconds allow developers to measure execution time and identify bottlenecks.
High Precision Timing
For even finer control, Python’s time module also provides nanoseconds using time.time_ns(). Example:
nanoseconds_since_epoch = time.time_ns()
print("Nanoseconds since Epoch:", nanoseconds_since_epoch)
This method is beneficial for scientific computations or high-performance applications that demand nanosecond accuracy.
By combining these approaches, you can build robust systems capable of measuring and processing time with unparalleled precision.
Get Time in Nanoseconds
Python introduced the time.time_ns() method in version 3.7, providing the ability to measure time with nanosecond precision. Unlike time.time(), which returns seconds as a floating-point number, time.time_ns() gives an integer value representing the number of nanoseconds since the Unix epoch (January 1, 1970).
Example Usage
Here’s a simple example to fetch the current time in nanoseconds:
import time
nanoseconds_since_epoch = time.time_ns()
print("Nanoseconds since Epoch:", nanoseconds_since_epoch)
Output might look like: 1705509123456789123.
Why Use Nanoseconds?
Nanosecond precision is essential in scenarios requiring extremely high accuracy and precision.
- High-Frequency Trading: In financial systems, where stock trades occur in fractions of a second, nanoseconds ensure timestamps are precise enough to manage and verify transactions.
- Performance Benchmarks: Developers use nanoseconds to measure code execution times with greater accuracy, especially in performance-critical applications.
- Scientific Research: Experiments requiring exact time intervals, such as quantum computing or particle physics, benefit from this precision.
- Real-Time Systems: Applications like robotics and industrial automation rely on precise timing for synchronization and decision-making.
Comparing with Other Methods
While time.time() gives precision up to milliseconds, time.time_ns() is significantly more accurate. For example:
import time
time_in_seconds = time.time()
time_in_nanoseconds = time.time_ns()
print("Time in Seconds:", time_in_seconds)
print("Time in Nanoseconds:", time_in_nanoseconds)
Output:
Time in Seconds: 1705509123.456789
Time in Nanoseconds: 1705509123456789123
This shows how time.time_ns() captures more detail, allowing you to differentiate events occurring in less than a millisecond.
Practical Use Cases
- Latency Measurement: In network systems, nanoseconds help measure and optimize data transmission times.
- Precise Event Ordering: Systems handling concurrent events can use nanoseconds to ensure accurate ordering, especially in databases or distributed systems.
- Gaming Engines: For smoother frame rendering and input response, nanosecond precision enables highly responsive gaming environments.
Limitations and Considerations
- Hardware Dependency: The actual precision depends on the underlying hardware. Some systems may not achieve true nanosecond accuracy.
- System Overhead: Frequent use of nanosecond measurements can add processing overhead. It’s best used only when the application demands extreme precision.
Nanosecond precision in Python empowers developers to tackle advanced challenges in high-stakes, performance-driven industries. Leveraging this feature can enhance the precision and effectiveness of your applications.
Additional Techniques for Working with Date and Time
Python’s datetime module offers versatile tools to handle date and time effectively. Beyond fetching the current date and time, several advanced techniques can help you handle timestamps, calculate durations, ensure calendar accuracy, and localize date formats.
1. Convert Date and Time to Timestamps
Timestamps are numerical representations of time, often used in databases, logs, or unique identifiers. Python’s timestamp() method converts a datetime object into a Unix timestamp.
Example:
from datetime import datetime
now = datetime.now()
timestamp = now.timestamp()
print("Timestamp:", timestamp)
Output:
Timestamp: 1705509123.456789
Key Points:
- The result is a floating-point number representing seconds since the Unix epoch (January 1, 1970).
- Timestamps are ideal for storing time data consistently across systems.
- They are frequently used in APIs, event logs, and performance benchmarking.
2. Calculate Time Differences
The timedelta class enables you to compute differences between two datetime objects.
Example:
from datetime import datetime, timedelta
now = datetime.now()
yesterday = now - timedelta(days=1)
difference = now - yesterday
print("Time Difference:", difference)
Output:
Time Difference: 1 day, 0:00:00
Practical Uses:
- Duration Tracking: Measure elapsed time for tasks or processes.
- Countdowns: Create countdowns for events or deadlines.
Date Adjustments: Easily add or subtract days, hours, or seconds from a given date.
next_week = now + timedelta(days=7)
print("Same time next week:", next_week)
3. Handle Leap Years
Leap years, occurring every four years, add an extra day in February (29th). Python’s calendar module helps verify leap years.
Example:
from calendar import isleap
year = 2024
print(f"Is {year} a leap year? {isleap(year)}")
Output:
Is 2024 a leap year? True
Why It’s Important:
- Ensures accuracy in scheduling, especially for yearly operations.
- Useful in financial applications calculating interest or payment schedules.
- Critical for algorithms dealing with date ranges.
4. Get ISO Format Dates
ISO 8601 is a standard for date-time formatting, commonly used in APIs and international applications. Python provides built-in support for ISO formatting.
Example:
from datetime import datetime
iso_format = datetime.now().isoformat()
print("ISO Format:", iso_format)
Output:
ISO Format: 2025-01-17T10:15:30.123456
Advantages:
- Standardization: Ensures consistency in data sharing across systems.
- Readability: Widely recognized by developers and systems worldwide.
- Time Zones: Easily supports UTC and offset values for global use.
5. Format Dates in Local Language
With the locale module, you can present dates and times in region-specific formats and languages.
Example:
from datetime import datetime
import locale
locale.setlocale(locale.LC_TIME, 'fr_FR') # Set to French
formatted_date = datetime.now().strftime("%A, %d %B %Y")
print("Date in French:", formatted_date)
Output:
Date in French: vendredi, 17 janvier 2025
Benefits:
- Multilingual Applications: Tailor your app’s user interface to specific regions.
- Region-Specific Reporting: Generate reports aligned with local conventions.
- Enhanced User Experience: Display information in a way familiar to users.
6. Additional Practical Techniques
Convert Strings to Datetime Objects
Parsing date strings is common in user inputs or data imports. Use datetime.strptime() to convert a date string into a datetime object.
from datetime import datetime
date_string = "17/01/2025"
date_object = datetime.strptime(date_string, "%d/%m/%Y")
print("Parsed Date:", date_object)
Round Dates to the Nearest Unit
Use timedelta to round dates or times to the nearest day, hour, or minute.
from datetime import datetime, timedelta
now = datetime.now()
rounded_to_hour = now.replace(minute=0, second=0, microsecond=0)
print("Rounded to Hour:", rounded_to_hour)
Handle Time Zones More Efficiently
For more robust timezone handling, use zoneinfo (Python 3.9+).
from datetime import datetime
from zoneinfo import ZoneInfo
now_utc = datetime.now(ZoneInfo("UTC"))
now_new_york = datetime.now(ZoneInfo("America/New_York"))
print("UTC Time:", now_utc)
print("New York Time:", now_new_york)
Also Read: How to Split a String in Python?
Conclusion
The datetime module in Python equips developers with a rich suite of functionalities for handling date and time effortlessly. Whether you need to fetch the current timestamp, format a date for readability, or work with international time zones, this module provides an intuitive and powerful approach. Its design supports both simple tasks like displaying the current time and more complex operations such as calculating date differences or converting times into standardized formats (e.g., ISO 8601).
With Python’s datetime tools, you can ensure accuracy across a wide variety of real-world applications. For example, developers can build systems that handle global scheduling, time-sensitive transactions, or performance tracking, all while ensuring data consistency and precision. The ability to manage different time zones, account for leap years, and perform high-precision time measurements means that Python is adaptable to diverse needs, whether for local or global applications.
By mastering Python’s datetime module, you simplify otherwise complex time-related tasks and enhance the reliability of your applications. These tools make it easier to handle time zones, date formatting, and scheduling, saving developers valuable time and effort. Start experimenting with these features today to improve the efficiency, reliability, and precision of your Python projects.